5. Putting it all together, the last two lines of our code!
my_shooter_game = ShooterGame() my_shooter_game.run()
The first line creates an object of type ShooterGame. This in turn automatically calls __init__ on the newly created object thus loading our world. The next line calls a method on our new object called ‘run’. Run is a method provided by the ShowBase class ShooterGame inheritied from. The run method starts the ‘game loop’, essentially updating the screen frame by frame, but it also handles tasks and events (we’ll see this later when we start adding, for example, keyboard controls to move your player around the world). That’s it! After all the preceding explanation, the bit of code that actually does something is really simple! …and there, you just saw, one of the many great benefits of Object Oriented programming!
6. Adding our Actor
The bit you have been waiting for!
For now, we are going to be using a model of SU27 Flanker aircraft. You can always change this to something else in the future (a space craft, a helicopter, whatever you desire) but it’s a bit too early to be discussing obtaining models, converting models, creating models and animating models. Yes, there’s a lot involved with models, and we’ll get to them in much greater detail in future issues.
You can download the flanker model using the links below. There are two files – the EGG file, and a texture file (remember, Panda3D models can be EGG or BAM files). Download both and save them into the same directory as your game:
(you may need to right-click and ‘save as’ depending on your operating system/browser)
Now update your code to look like this:
from direct.showbase.ShowBase import ShowBase class ShooterGame(ShowBase): def __init__(self): ShowBase.__init__(self) self.world = self.loader.loadModel("world.bam") self.world.reparentTo(self.render) self.player = self.loader.loadModel("alliedflanker.egg") self.player.setPos(20,20,65) self.player.setH(225) self.player.reparentTo(self.render) my_shooter_game = ShooterGame() my_shooter_game.run()
Run your game and move your camera around as you did in Issue 1. You should find your player hovering over the terrain. Like this:
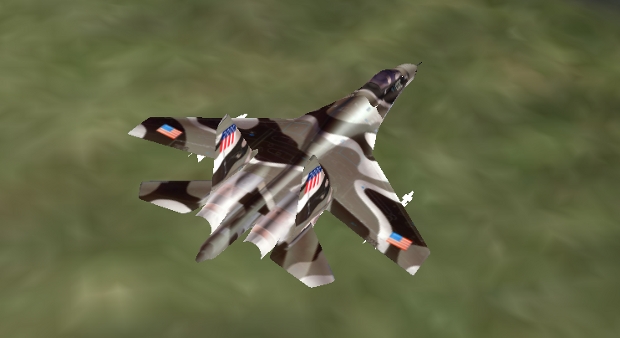
In true MGF style, we’re not going to discuss this new code right now. We’ll open Issue 3 with a re-cap and explanation!
7. Wrap up and Summary
Yeah, we’re bad. We started out with the aim of adding your player – but we knew it required some tricky concepts and explanation and we kinda just hit you with them. The good news is it doesn’t really get any more difficult than this! If you can get your head around classes, objects, methods and variables you will have no problems in future issues!
We’ll leave you with an uber short summary of what you have just encountered (plus a little bit more!). It doesn’t have to make full sense now, but we feel the below summaries capture the whole idea of object oriented as succinctly as possible:
- Objects have Behaviour, Identity and State
- Classes offer Inheritance, Encapsulation and Polymorphism
…we’ll hit on each of the above again real soon!